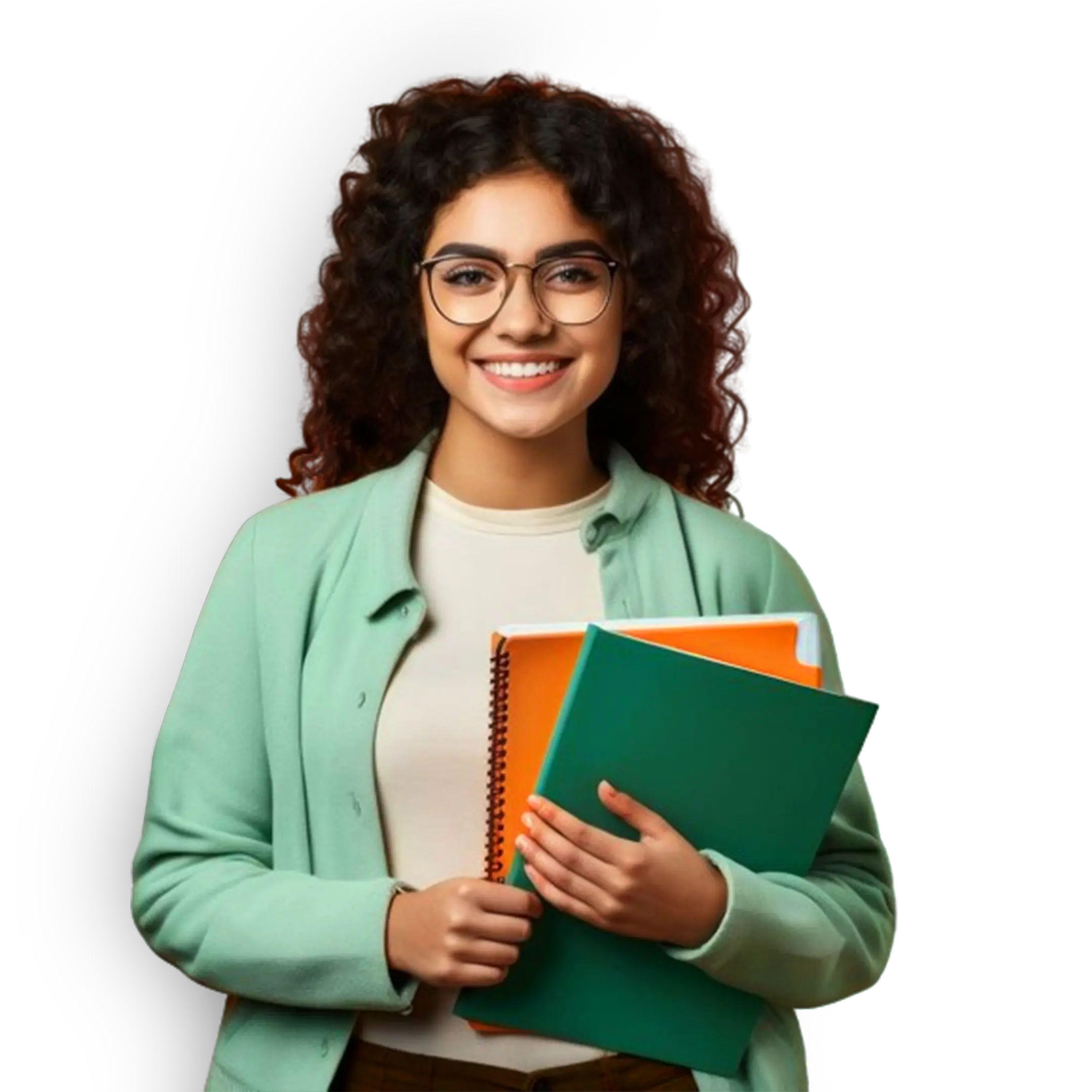
We provide a comprehensive Java full stack training program spanning 7 months, integrating key technologies such as HTML, CSS, Bootstrap, JavaScript, jQuery, AJAX, MySQL, and Spring framework. Participants gain proficiency in both front-end and back-end development, leveraging Java for building robust web applications. The course emphasizes practical learning through real-world projects, assignments, and dedicated sessions for interview preparation, ensuring students acquire hands-on experience and industry-relevant skills. Located in Trivandrum, Trinity Technologies stands out as Kerala's foremost information technology training institute, dedicated to equipping aspiring developers with the expertise required for successful careers in Java web development.
01
Overview and History
Features of Java
C++ vs Java
Setting the path for Java
02
JDK, JRE and JVM
JVM Memory Management
Internal details of JVM
Unicode system
03
Basic Syntax
Operators and Expressions
Single line comments
Multi-line comments
04
Variable declaration and initialization
Naming conventions
Types of variables: Local variables, Instance variables, Static variables
Scope and memory allocation of variables
05
Operators and Operands
Arithmetic Operators
Assignment Operators
Comparison Operators
Logical Operators
Unary Operators
06
Integral data types
Floating point Data Type
Boolean Data Type
Char Data Type
Reference Data Types
07
Simple if statements
if else statements
if elseif else statements
Nested if statements
Switch statements
08
for loop
for each loop
while loop
do...while loop
Break statement
Continue statement
09
Naming conventions in Java
Declaring a Class
Object Declaration and Initialization
Life cycle of an object
Anonymous object in Java
10
Declaring a package in a company project
Package naming conventions
Subpackages
User-defined and built-in packages
Importing packages in Java
11
Methods in Java
Use of a method in Java
Method declaration, Method signature
Pre-defined methods
User-defined methods
Instance methods
Static methods
Calling a method
Java main method
Return type in Java
12
Default constructor
Parameterized constructors
Java constructor overloading
Constructor chaining
Copy constructor in Java
13
Access Modifiers
Deafult modifiers
Private modifiers
Protected modifiers
Public modifiers
Non-access modifiers
Abstract modifiers
Final modifiers
Native modifiers
strictfp modifiers
Synchronized modifiers
Transient modifiers
Volatile modifiers
14
Static Keyword
Static variable
Static method
Static block
Instance block
Static nested class block
15
Final keyword
Final variable
Final method
Final class
Best practices for using final
16
Inner Class in Java
Properties of inner class
Instantiating inner class
Normal inner class
Method local inner class
Anonymous inner class
Static nested inner class
17
super keyword
Calling of superclass instance variable
Super class constructor
Super class method
this keyword
Calling of current class constructor and method
18
Java OOP Concept
Java Class and Object
Encapsulation
Inheritance
Polymorphism
Abstraction
19
How to acheive encapsulation
Data Hiding
Tightly encapsulated class
Getter and setter methods in Java
Naming conventions of getter and setter methods
20
Inheritance in Java
Is-A Relationship
Aggregation and composition
Types of inheritance
21
Polymorphism in Java
Compile time polymorphism
Runtime polymorphism
Static and dynamic binding
Method Overloading
Method Overriding
Covariant Return Type
22
Heap Memory
Reference Counting Vs. Mark-and-sweep
Garbage Collector
Tuning and monitoring
Advantages of garbage collection
Best practices
23
Streams Basics
InputStream and OutputStream
File I/O
Buffered streams
ByteArray streams
Character array streams
Data streams
Filter streams
Object streams
Console
FilePermissionWriter, Reader, FileWriter, FileReader
PrintStream
Pushback stream
StringWriter
StringReader
PipedWriter
PipedReader
FilterWriter, FilterReader, File FileDescriptor, RandomAccessFile, and java.util.Scanner
24
Collections Framework
List
Set
Sorted set
Queue
Deque
Map
Iterator
List iterator
Enumeration
ArrayList
LinkedList
HashSet
LinkedHashSet
TreeSet
ArrayDeque
PriorityDeque
EnumSet
AbstractCollection
AbstractList
AbstractQueue
AbstractSet
AbstractSequentialList
Map
Map Entry
SortedMap
NavigableMap
MapHashMap
LinkedHashMap
TreeMap
IdentityHashMap
WeakHashMap
EnumMap
Comparator, Random Access interfaces as well as observable class
25
Serialization in Java
Deserialization
Transient fields
Best practices
26
Exception Handling in Java
Try-catch block
Multiple Catch Block
Nested try block
Finally block
Throw Keyword
Throws Keyword
Throw vs Throws, Final vs Finally vs Finalize
Exception Handling with Method Overriding Java Custom Exceptions
27
Built-in annotaions
@Override
@SuppressWarnings
@Deprecated
@Target
@Retention
@Inherited
@Documented
Custom annotations
Types of annotations
28
Reflection API
NewInstance() & Determining the class object
Javap tool
Creating javap tool
Creating applet viewer
Accessing private method from outside the class
29
Java Array
Single dimensional array
Multidimensional array
Array declaration
Array instantiation
initialization of Java array
Passing array to a method
Anonymous array in Java
Cloning an array in Java
30
Java Strings,
Immutable String
String Comparison, String concatenation
Substring
StringBuffer class
StringBuilder class
toString method
StringTokenizer class
31
Java multithreading
Multithreading life cycle of a thread creating
Thread scheduler
Sleeping a thread
Start a thread twice
Calling run() method
Joining a thread
Naming a thread
Thread priority,
Daemon thread
Thread pool
Thread group
Shutdownhook
Java Synchronization: synchronized method, synchronized block, static synchronization
Deadlock
Inter-thread Communication
Interrupting Thread
32
JDBC Drivers
Steps to connect to Database
Connectivity with Oracle
Connectivity with MySQL
Connectivity with Access without DSN
DriverManager
Types of JDBC statements: Statement, Prepared statement, Callable statement
Database Metadata, Resultset Metadata
ResultSet, types of ResultSet,
Storing image, Retrieving image
Storing file, Retrieving file, Stored procedures, and functions
Transaction Management
Batch Processing
JDBC New Features, Mini Project, and interview questions.
33
Agile model
Advantages, and Disadvantages of Agile model
Agile versus Waterfall method
Scrum
Scrum Master
Flow of Agile Implementation
Sprint
Burn down Charts
34
Singleton Object
Singleton design pattern with Serialization
Factory Pattern
Abstract Factory.
35
MySQL Introduction
MySQL Create Database
MySQL Queries
MySQL DDL Statements
MySQL DML Statements
MySQL Join Queries
MySQL Conditions
MySQL Insert Data
MySQL Get Last ID
MySQL OrderBy
MySQL Limit Data
MySQL Between
MySQL Join
01
What is Spring
How Spring fits into the Enterprise world
Spring Modules
02
Core Container
Introduction to IOC
Types of DI
Setter VS Constructor
Collection DI
Bean Inheritance
Collection Merging
Inner Beans
Bean Aliasing
Bean Scopes
Inner Beans
Null String
Bean Auto wiring
03
P-Namespace
C-Namespace
Dependency Check
Depends On
Factory Beans
Static Factory Method
Instance Factory Method
Bean Lifecycle
04
AOP Concepts
Programmatic VS Declarative AOP
Programmatic AOP
Types of Advices
Types of Pointcuts
Working with proxies
Declarative AOP
Using AOP 2.0 Config element
OGNL Expressions
05
Spring Transaction Management
AOP 2.0 Configuration driven Transaction Management
Aspect J annotation based Transaction Management
06
What is DAO pattern?
Ways to implement Spring DAO
Choosing an approach for JDBC database access
JDBC Template
Executing Statements
SimpleJDBCInsert
Running Queries
SQL Parameters
Mapping SQL Operations as Subclasses
07
Spring 3.0 features
Introduction to Spring MVC
Handler Mapping
Controllers
Validations
Handler Interceptors
Views
Form tags
01
Basic concepts and History
Client-Side technologies
Server-Side technologies
Web Development Tools and IDEs
Web standards and protocols
02
HTML Basics
Options for writing HTML
Basic tags and document structure
Default text editor
HTML elements
Saving HTML document
03
Types of tags
Document Strucutre
`<html>` tag
`<head>` tag
`<title>` tag
`<meta>` tag
`<body>` tag
04
Heading Tags: `<h1>` to `<h6>`
Paragragh tag
Line Breaks
HTML Comments
Text formatting tags
05
Global Attributes
Event Attributes
Form Attributes
Input Attributes
Media Attributes
Style Attributes
Accessibility Attributes
06
Non-breaking space
Horizontal Rule
Special Characters
Fonts and Text Styling
Font Families
`<font>` tag
07
Images: `<img>` tag
Hyperlinks: `<a>` tag
Video: `<video>` tag
Audio: `<audio>` tag
Embedding extrenal content in a HTML page: `<iframe>` tag
08
Tables: `<table>`, `<thead>`, `<tbody>`, `<tr>`, `<th>`,`<td>` tags
Ordered Lists
Unordered Lists
Definition Lists
Nested Lists
09
Form Elements
Form Attributes
Input Types / Form Controls
Form Layouts
01
CSS Introduction
CSS Syntax
CSS Inline Method
CSS Internal Styling
CSS External Styling
02
Element Selectors
Class Selectors
ID Selectors
Attribute Selectors
Universal Selectors
Grouping Selectors
Psudeo-Elements
Psuedo Classes
03
Font Properties
Text color and background
Text decoration
Alignment and transformation
Line-height and spacing
Text Shadow
Overflow and wrapping
04
Content area
Padding
Border
Outline
Padding
Dimensions
Box-sizing
05
Positioning elements
CSS Display
CSS Floating
CSS Overflow
CSS Grid system
Responsive techniques
Centering elements
06
Box Shadows
Text Shadows
Opacity and transparency
Transforms
Gradient backgrounds
Filter effects
Clip path
Transitions
Animations
07
Styling Links
Image Gallery
CSS Navigation Bar
Hover Effects
Focus styles
Active state
08
Syntax and Structure
Media types
Media features
Combining media features
Viewport units
Breakpoints and respononsive design
Mobile First and Desktop First approach
Nested media queries
01
Overview and History
Why Bootstrap
Methods for including Bootstrap in a web page
Responsive web pages
02
BS5 Containers
BS5 Grid System
BS5 Typography
BS5 Tables
BS5 Colors
BS5 Images
03
BS5 Alert
BS5 Jumbotron
BS5 Buttons
BS5 Pagination
BS5 List Groups
BS5 Spinners
BS5 Cards
BS5 Dropdown
BS5 Collapse
BS5 Navbar
BS5 Carousel
BS5 Modal
04
Bootstrap Grid System
BS5 Stacked / Horizontal
BS5 Grid xsmall, small, medium, large, xlarge, xxl
Bootsrap Grid Examples
05
Form Layouts
Form Controls
Form Validation
Input Groups
Form Text
Checkboxes and radios
Form attributes
Form layout utilities
01
JS Overview and History
JS Syntax
JS Statements
JS Comments
02
Var, let and const
Declaration and Initialization
Scope
Hoisting
03
String
Number
Bigint
Boolean
Undefined
Null
Symbol
Object
Array
04
Operators and Operands
Arithmetic Operators
Assignment Operators
Comparison Operators
Logical Operators
05
if Statement
if else Statement
if else if else Statement
Switch statement
for Loop
while Loop
do...while Loop
Break and Continue Statements
Nested Control Structures
06
Function Declaration and Invocation
Function Parameters and Arguments
Return Statement
Function scope and closure
Default parameters
JS String Functions
JS Array Functions
07
Event Handling Basics
Inline event Handlers
Event Object
Mouse Events
Keyboard Events
Form Events
Input Events
Window and Document Events
08
Basic Validation Techniques
Event binding and handling
Validation functions
Error Messaging
Validation state management
Real-time feedback
01
AJAX Overview
Advantages of AJAX
AJAX Architecture
AJAX XMLHttp
Creating an XMLHttp request
Sending and receiving data with XMLHttp
02
Making asynchronous requests
GET vs POST requests
Handling request headers
Handling server responses
Parsing response data
Error handling in AJAX
03
Setting up a PHP backend for AJAX
Sending data from AJAX to PHP
Processing AJAX requests in PHP
04
Connecting to MySQL database with PHP
Retrieving data with AJAX from MySQL via PHP
Updating MySQL database using AJAX and PHP
05
Implementing AJAX in web applications
Examples of real-world AJAX usage
Best practices and considerations
01
What's jQuery
History and Evolution
Benefits Of using jQuery
Core Features and Capabilities
02
Basic Syntax
Using jQuery selectors and methods
Chaining methods
03
Overview of selectors in jQuery
CSS style selectors
Attribute selectors
Hierarchy and descendant selectors
Advanced selectors and filtering
04
Implementing AJAX in web applications
Examples of real-world AJAX usage
Best practices and considerations
05
Showing and hiding elements
Fading effects
Sliding effects
06
Basic Animations
Custom animations with jQuery
Using easing functions
07
Understanding callback functions in jQuery
Using callbacks with animations and effects
Chaining animations with callbacks
08
Manipulating HTML content with jQuery
Creating and modifying DOM elements
Form manipulation with jQuery
01
Basics
Introduction to PHPMyAdmin
Working with graphical console
Database Backup
Database Restore
02
MySQL database creation
MySQL DDL Statements
Creating Tables
Altering Tables
Dropping Tables
03
MySQL Queries
MySQL DML Statements
MySql Insert Data
MySql Update Data
MySQL Delete Data
MySQL get last ID
04
MySQL Join Queries
MySQL Join Types
MySQL Conditions
MySQL Order By
MySQL Limit Data
MySQL Between
Trinity Technologies in Trivandrum offers an extensive Java training and certification program tailored to equip learners with robust programming skills and industry-relevant expertise. This course is ideal for both beginners and professionals aiming to advance their careers in software development. Through a combination of hands-on projects and expert-led instruction, students gain comprehensive knowledge of Java fundamentals, object-oriented programming, data structures, algorithms, and advanced Java frameworks like Spring and Hibernate. Trinity Technologies ensures that participants are thoroughly prepared to achieve their Java certification, making it a preferred choice for aspiring developers in the region.
Java remains one of the most widely used and versatile programming languages in the software industry. Earning a Java certification can significantly boost career prospects, as companies across the globe seek skilled Java developers to build, maintain, and optimize their software applications. Whether you are looking to start your journey in programming or enhance your existing skills, Trinity Technologies provides a structured pathway to achieve your goals.
Trinity Technologies has established itself as a premier IT training institute in Trivandrum, known for its rigorous curriculum and experienced faculty. The Java training program is meticulously designed to cover both the basics and advanced aspects of Java programming, ensuring that students not only learn the language but also understand how to apply it in real-world scenarios.
The faculty at Trinity Technologies comprises seasoned professionals who bring years of industry experience into the classroom. These instructors are well-versed in the latest developments in Java and related technologies, providing students with insights that go beyond textbooks. Their practical approach to teaching ensures that students grasp complex concepts with ease and are well-prepared for the Java certification exam.
Trinity Technologies boasts modern infrastructure with fully equipped labs, providing students with the tools necessary for immersive learning. The training environment includes the latest software and development tools, enabling students to practice coding, debug applications, and work on real-time projects. This hands-on experience is crucial for developing the practical skills required in today's competitive job market.
The Java training program at Trinity Technologies covers a wide array of topics essential for mastering the language. The curriculum is aligned with industry standards and includes the following key areas:
Core Java Fundamentals: Introduction to Java, understanding Java syntax, and exploring object- oriented programming concepts.
Advanced Java Programming: In-depth coverage of multithreading, exception handling, I/O streams, and network programming.
Java Frameworks: Hands-on training with popular frameworks like Spring and Hibernate, focusing on building scalable and efficient enterprise applications.
Database Integration: Learning how to work with databases using JDBC and Hibernate ORM for data persistence.
Web Development: Developing dynamic web applications using Servlets, JSP, and integrating front-end technologies.
Testing and Debugging: Best practices for testing Java applications, debugging techniques, and performance optimization.
Trinity Technologies places a strong emphasis on practical learning, with students working on real- world projects that simulate industry environments. This approach allows learners to apply theoretical knowledge to practical scenarios, enhancing their problem-solving skills and preparing them for real challenges in the software development field.
To ensure that students are ready for the Java certification exam, Trinity Technologies conducts regular assessments and mock tests. These evaluations help identify areas for improvement and provide students with the confidence needed to excel in the certification process.
Trinity Technologies is committed to helping students transition from learning to employment. The institute offers comprehensive career guidance, including resume building, interview preparation, and job search strategies. Additionally, their strong industry connections provide students with access to job opportunities in top IT companies.
Obtaining a Java certification is more than just a credential, it is a stepping stone to a successful career in software development. Here's why earning a Java certification from Trinity Technologies is essential:
Industry Recognition: Java certification is recognized globally, validating your skills and making you a desirable candidate for software development roles.
Career Advancement: Whether you"re a beginner or an experienced developer, a Java certification can open doors to advanced positions such as software engineer, application developer, or system architect.
Foundation for Future Learning: Java is a foundational language that sets the stage for learning other programming languages and technologies, making it easier to expand your skillset.
Increased Earning Potential: Certified Java professionals often command higher salaries due to their proven expertise and ability to contribute effectively to software projects.
Global Opportunities: Java is used worldwide, and certification provides you with the flexibility to pursue career opportunities in any region.
Trinity Technologies in Trivandrum is more than just a training institute. It is a gateway to a successful career in software development. The Java training program is comprehensive, up-to-date, and designed to equip students with the skills they need to excel in the fast-paced world of IT. With experienced faculty, cutting-edge infrastructure, and a commitment to student success, Trinity Technologies stands out as a leader in Java training.
By choosing Trinity Technologies for your Java training, you're not just earning a certification, you're investing in your future. The knowledge and skills you acquire here will form the foundation for a rewarding career in software development, opening up a world of opportunities.
What is Java?
Java is a high-level, object-oriented programming language developed by Sun Microsystems (now owned by Oracle) in 1995. It is designed to be platform-independent, meaning that code written in Java can run on any device that has a Java Virtual Machine (JVM).
What is Java Virtual Machine (JVM)?
The JVM is an abstract computing machine that enables a computer to run Java programs. It converts Java bytecode into machine code so that it can be executed on any device. The JVM provides platform independence, as Java code can run on any device that has a compatible JVM.
What are the prerequisites for enrolling in this Java course?
No prior programming experience is required. The course is designed for beginners, though basic computer literacy and problem-solving skills will be helpful. A keen interest in programming and a willingness to learn are the main prerequisites.
What topics will be covered in the Java course?
The course will cover key Java concepts, including:
Basics of Java syntax and programming structure
Variables, data types, and operators
Control flow (loops and conditionals)
Object-oriented programming
Exception handling
File I/O
Collections framework (lists, sets, maps)
Java programming best practices
What materials and resources will I need for the course?
The institute will provide all necessary course materials, including textbooks and online resources. You will need to bring a laptop (if available else one will be provided) with Java Development Kit (JDK) installed, which will be guided by the instructor before the course starts.
What if I miss a class?
If you miss a class, you can catch up by reviewing the course materials and notes provided. You may also be able to arrange a make-up session with the instructor or seek additional help during office hours.
How can I get help if I have questions or need clarification?
You can ask questions during class, and the instructor will be available for additional support. Office hours or scheduled help sessions will also be provided to address any further questions or difficulties.
Will I receive a certificate upon completion of the course?
Yes, upon successful completion of the course, you will receive a certificate of completion from the institute. This certificate can help demonstrate your Java programming skills to potential employers.
What is Spring in Java?
Spring is a comprehensive framework for building enterprise-level applications in Java. It provides infrastructure support for developing Java applications, including dependency injection, aspect-oriented programming (AOP), and transaction management. Spring simplifies the development of enterprise applications by providing a robust platform for managing various layers of an application, from the web layer to the database layer.
How do I enroll in the Java course?
To enroll, visit the institute's website or contact the admissions office directly. You will need to complete a registration form and a free demo class will be provided. Enrollment procedures will be provided by the institute.