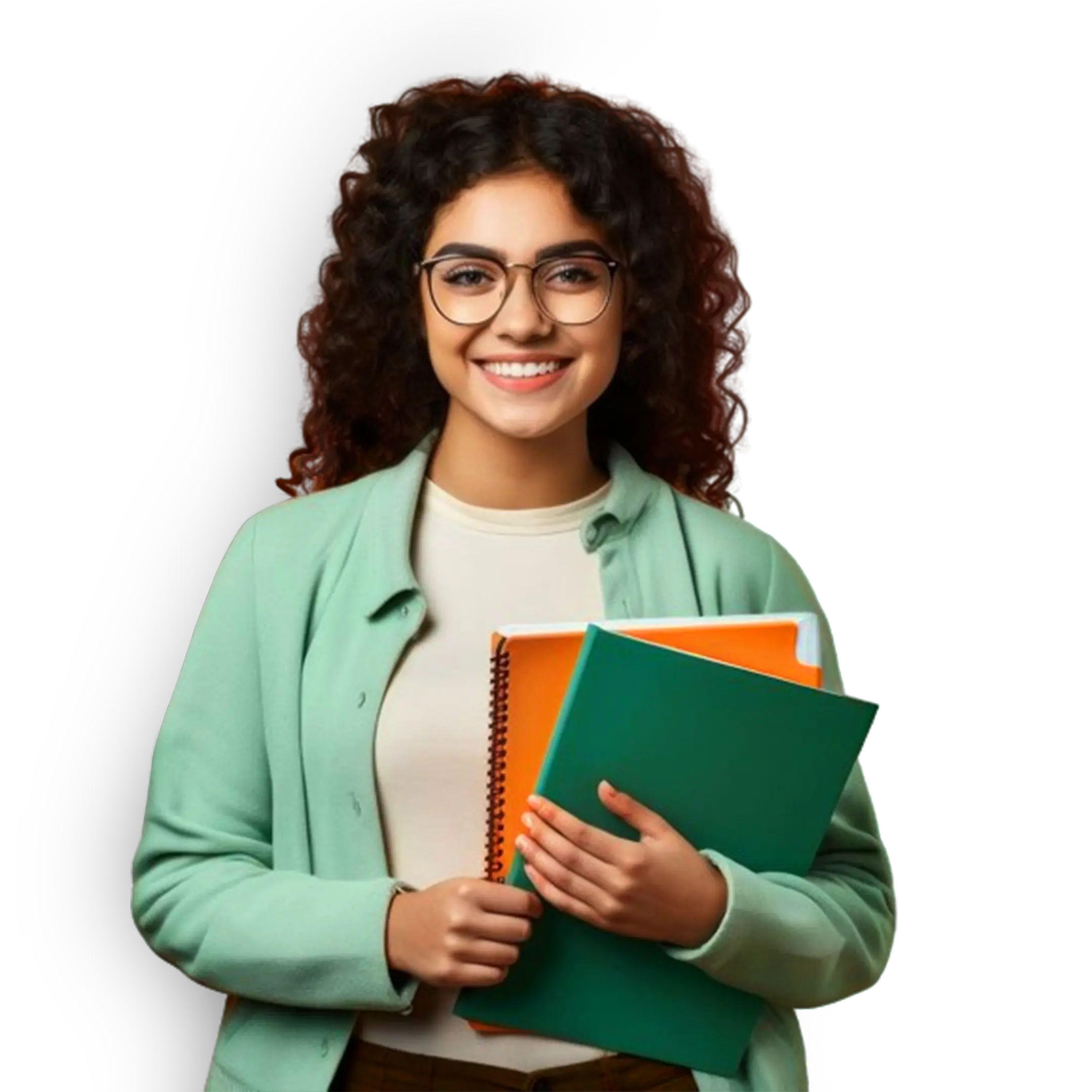
We offer a rigorous Java full stack training program spanning 7 months, integrating essential technologies such as HTML, CSS, Bootstrap, JavaScript, jQuery, AJAX, MySQL, and JavaFX. Participants engage in comprehensive training covering both front-end and back-end development using Java, with a particular emphasis on JavaFX for building rich client applications. The curriculum is designed to provide hands-on experience through real-world projects, assignments, and focused interview preparation sessions, ensuring students acquire practical skills and proficiency in industry-standard tools and frameworks. Located in Trivandrum, Trinity Technologies is renowned as Kerala's premier information technology training institute, dedicated to equipping aspiring developers with the expertise needed for successful careers in Java full stack development, including JavaFX for desktop and client-side applications.
01
Overview and History
Features of Java
C++ vs Java
Setting the path for Java
02
JDK, JRE and JVM
JVM Memory Management
Internal details of JVM
Unicode system
03
Basic Syntax
Operators and Expressions
Single line comments
Multi-line comments
04
Variable declaration and initialization
Naming conventions
Types of variables: Local variables, Instance variables, Static variables
Scope and memory allocation of variables
05
Operators and Operands
Arithmetic Operators
Assignment Operators
Comparison Operators
Logical Operators
Unary Operators
06
Integral data types
Floating point Data Type
Boolean Data Type
Char Data Type
Reference Data Types
07
Simple if statements
if else statements
if elseif else statements
Nested if statements
Switch statements
08
for loop
for each loop
while loop
do...while loop
Break statement
Continue statement
09
Naming conventions in Java
Declaring a Class
Object Declaration and Initialization
Life cycle of an object
Anonymous object in Java
10
Declaring a package in a company project
Package naming conventions
Subpackages
User-defined and built-in packages
Importing packages in Java
11
Methods in Java
Use of a method in Java
Method declaration, Method signature
Pre-defined methods
User-defined methods
Instance methods
Static methods
Calling a method
Java main method
Return type in Java
12
Default constructor
Parameterized constructors
Java constructor overloading
Constructor chaining
Copy constructor in Java
13
Access Modifiers
Deafult modifiers
Private modifiers
Protected modifiers
Public modifiers
Non-access modifiers
Abstract modifiers
Final modifiers
Native modifiers
strictfp modifiers
Synchronized modifiers
Transient modifiers
Volatile modifiers
14
Static Keyword
Static variable
Static method
Static block
Instance block
Static nested class block
15
Final keyword
Final variable
Final method
Final class
Best practices for using final
16
Inner Class in Java
Properties of inner class
Instantiating inner class
Normal inner class
Method local inner class
Anonymous inner class
Static nested inner class
17
super keyword
Calling of superclass instance variable
Super class constructor
Super class method
this keyword
Calling of current class constructor and method
18
Java OOP Concept
Java Class and Object
Encapsulation
Inheritance
Polymorphism
Abstraction
19
How to acheive encapsulation
Data Hiding
Tightly encapsulated class
Getter and setter methods in Java
Naming conventions of getter and setter methods
20
Inheritance in Java
Is-A Relationship
Aggregation and composition
Types of inheritance
21
Polymorphism in Java
Compile time polymorphism
Runtime polymorphism
Static and dynamic binding
Method Overloading
Method Overriding
Covariant Return Type
22
Heap Memory
Reference Counting Vs. Mark-and-sweep
Garbage Collector
Tuning and monitoring
Advantages of garbage collection
Best practices
23
Streams Basics
InputStream and OutputStream
File I/O
Buffered streams
ByteArray streams
Character array streams
Data streams
Filter streams
Object streams
Console
FilePermissionWriter, Reader, FileWriter, FileReader
PrintStream
Pushback stream
StringWriter
StringReader
PipedWriter
PipedReader
FilterWriter, FilterReader, File FileDescriptor, RandomAccessFile, and java.util.Scanner
24
Collections Framework
List
Set
Sorted set
Queue
Deque
Map
Iterator
List iterator
Enumeration
ArrayList
LinkedList
HashSet
LinkedHashSet
TreeSet
ArrayDeque
PriorityDeque
EnumSet
AbstractCollection
AbstractList
AbstractQueue
AbstractSet
AbstractSequentialList
Map
Map Entry
SortedMap
NavigableMap
MapHashMap
LinkedHashMap
TreeMap
IdentityHashMap
WeakHashMap
EnumMap
Comparator, Random Access interfaces as well as observable class
25
Serialization in Java
Deserialization
Transient fields
Best practices
26
Exception Handling in Java
Try-catch block
Multiple Catch Block
Nested try block
Finally block
Throw Keyword
Throws Keyword
Throw vs Throws, Final vs Finally vs Finalize
Exception Handling with Method Overriding Java Custom Exceptions
27
Built-in annotaions
@Override
@SuppressWarnings
@Deprecated
@Target
@Retention
@Inherited
@Documented
Custom annotations
Types of annotations
28
Reflection API
NewInstance() & Determining the class object
Javap tool
Creating javap tool
Creating applet viewer
Accessing private method from outside the class
29
Java Array
Single dimensional array
Multidimensional array
Array declaration
Array instantiation
initialization of Java array
Passing array to a method
Anonymous array in Java
Cloning an array in Java
30
Java Strings,
Immutable String
String Comparison, String concatenation
Substring
StringBuffer class
StringBuilder class
toString method
StringTokenizer class
31
Java multithreading
Multithreading life cycle of a thread creating
Thread scheduler
Sleeping a thread
Start a thread twice
Calling run() method
Joining a thread
Naming a thread
Thread priority,
Daemon thread
Thread pool
Thread group
Shutdownhook
Java Synchronization: synchronized method, synchronized block, static synchronization
Deadlock
Inter-thread Communication
Interrupting Thread
32
JDBC Drivers
Steps to connect to Database
Connectivity with Oracle
Connectivity with MySQL
Connectivity with Access without DSN
DriverManager
Types of JDBC statements: Statement, Prepared statement, Callable statement
Database Metadata, Resultset Metadata
ResultSet, types of ResultSet,
Storing image, Retrieving image
Storing file, Retrieving file, Stored procedures, and functions
Transaction Management
Batch Processing
JDBC New Features, Mini Project, and interview questions.
33
Agile model
Advantages, and Disadvantages of Agile model
Agile versus Waterfall method
Scrum
Scrum Master
Flow of Agile Implementation
Sprint
Burn down Charts
34
Singleton Object
Singleton design pattern with Serialization
Factory Pattern
Abstract Factory.
35
MySQL Introduction
MySQL Create Database
MySQL Queries
MySQL DDL Statements
MySQL DML Statements
MySQL Join Queries
MySQL Conditions
MySQL Insert Data
MySQL Get Last ID
MySQL OrderBy
MySQL Limit Data
MySQL Between
MySQL Join
01
Basic Syntax
Idioms
Coding Conventions
02
Basic Types
Packages
Control Flow
Returns and Jumps
03
Classes and Inheritance
Properties and Fields
Interfaces
Visibility Modifiers
Extensions
Data Classes
Generics
Nested Classes
Enum Classes
Objects
Delegation
Delegated Properties
04
Functions
Lamdas
Event Handling with Lambdas
Inline functions
05
Destructuring Declarations
Collections
Ranges
Type Checks and Casts
This expressions
Equality
Operator overloading
Null Safety
Exceptions
Annotations
Reflection
Type-Safe Builders
Dynamic Type
06
Interoperability
Calling Java from Kotlin
Calling Kotlin from Java
07
Documenting Kotlin Code
Using Maven
Using Ant
Using Gradle
Kotlin and OSGi
01
Basic concepts and History
Client-Side technologies
Server-Side technologies
Web Development Tools and IDEs
Web standards and protocols
02
HTML Basics
Options for writing HTML
Basic tags and document structure
Default text editor
HTML elements
Saving HTML document
03
Types of tags
Document Strucutre
`<html>` tag
`<head>` tag
`<title>` tag
`<meta>` tag
`<body>` tag
04
Heading Tags: `<h1>` to `<h6>`
Paragragh tag
Line Breaks
HTML Comments
Text formatting tags
05
Global Attributes
Event Attributes
Form Attributes
Input Attributes
Media Attributes
Style Attributes
Accessibility Attributes
06
Non-breaking space
Horizontal Rule
Special Characters
Fonts and Text Styling
Font Families
`<font>` tag
07
Images: `<img>` tag
Hyperlinks: `<a>` tag
Video: `<video>` tag
Audio: `<audio>` tag
Embedding extrenal content in a HTML page: `<iframe>` tag
08
Tables: `<table>`, `<thead>`, `<tbody>`, `<tr>`, `<th>`,`<td>` tags
Ordered Lists
Unordered Lists
Definition Lists
Nested Lists
09
Form Elements
Form Attributes
Input Types / Form Controls
Form Layouts
01
CSS Introduction
CSS Syntax
CSS Inline Method
CSS Internal Styling
CSS External Styling
02
Element Selectors
Class Selectors
ID Selectors
Attribute Selectors
Universal Selectors
Grouping Selectors
Psudeo-Elements
Psuedo Classes
03
Font Properties
Text color and background
Text decoration
Alignment and transformation
Line-height and spacing
Text Shadow
Overflow and wrapping
04
Content area
Padding
Border
Outline
Padding
Dimensions
Box-sizing
05
Positioning elements
CSS Display
CSS Floating
CSS Overflow
CSS Grid system
Responsive techniques
Centering elements
06
Box Shadows
Text Shadows
Opacity and transparency
Transforms
Gradient backgrounds
Filter effects
Clip path
Transitions
Animations
07
Styling Links
Image Gallery
CSS Navigation Bar
Hover Effects
Focus styles
Active state
08
Syntax and Structure
Media types
Media features
Combining media features
Viewport units
Breakpoints and respononsive design
Mobile First and Desktop First approach
Nested media queries
01
Overview and History
Why Bootstrap
Methods for including Bootstrap in a web page
Responsive web pages
02
BS5 Containers
BS5 Grid System
BS5 Typography
BS5 Tables
BS5 Colors
BS5 Images
03
BS5 Alert
BS5 Jumbotron
BS5 Buttons
BS5 Pagination
BS5 List Groups
BS5 Spinners
BS5 Cards
BS5 Dropdown
BS5 Collapse
BS5 Navbar
BS5 Carousel
BS5 Modal
04
Bootstrap Grid System
BS5 Stacked / Horizontal
BS5 Grid xsmall, small, medium, large, xlarge, xxl
Bootsrap Grid Examples
05
Form Layouts
Form Controls
Form Validation
Input Groups
Form Text
Checkboxes and radios
Form attributes
Form layout utilities
01
JS Overview and History
JS Syntax
JS Statements
JS Comments
02
Var, let and const
Declaration and Initialization
Scope
Hoisting
03
String
Number
Bigint
Boolean
Undefined
Null
Symbol
Object
Array
04
Operators and Operands
Arithmetic Operators
Assignment Operators
Comparison Operators
Logical Operators
05
if Statement
if else Statement
if else if else Statement
Switch statement
for Loop
while Loop
do...while Loop
Break and Continue Statements
Nested Control Structures
06
Function Declaration and Invocation
Function Parameters and Arguments
Return Statement
Function scope and closure
Default parameters
JS String Functions
JS Array Functions
07
Event Handling Basics
Inline event Handlers
Event Object
Mouse Events
Keyboard Events
Form Events
Input Events
Window and Document Events
08
Basic Validation Techniques
Event binding and handling
Validation functions
Error Messaging
Validation state management
Real-time feedback
01
AJAX Overview
Advantages of AJAX
AJAX Architecture
AJAX XMLHttp
Creating an XMLHttp request
Sending and receiving data with XMLHttp
02
Making asynchronous requests
GET vs POST requests
Handling request headers
Handling server responses
Parsing response data
Error handling in AJAX
03
Setting up a PHP backend for AJAX
Sending data from AJAX to PHP
Processing AJAX requests in PHP
04
Connecting to MySQL database with PHP
Retrieving data with AJAX from MySQL via PHP
Updating MySQL database using AJAX and PHP
05
Implementing AJAX in web applications
Examples of real-world AJAX usage
Best practices and considerations
01
What's jQuery
History and Evolution
Benefits Of using jQuery
Core Features and Capabilities
02
Basic Syntax
Using jQuery selectors and methods
Chaining methods
03
Overview of selectors in jQuery
CSS style selectors
Attribute selectors
Hierarchy and descendant selectors
Advanced selectors and filtering
04
Implementing AJAX in web applications
Examples of real-world AJAX usage
Best practices and considerations
05
Showing and hiding elements
Fading effects
Sliding effects
06
Basic Animations
Custom animations with jQuery
Using easing functions
07
Understanding callback functions in jQuery
Using callbacks with animations and effects
Chaining animations with callbacks
08
Manipulating HTML content with jQuery
Creating and modifying DOM elements
Form manipulation with jQuery
01
Basics
Introduction to PHPMyAdmin
Working with graphical console
Database Backup
Database Restore
02
MySQL database creation
MySQL DDL Statements
Creating Tables
Altering Tables
Dropping Tables
03
MySQL Queries
MySQL DML Statements
MySql Insert Data
MySql Update Data
MySQL Delete Data
MySQL get last ID
04
MySQL Join Queries
MySQL Join Types
MySQL Conditions
MySQL Order By
MySQL Limit Data
MySQL Between
Trinity Technologies in Trivandrum offers a comprehensive JavaFX training and certification program designed to empower developers with the skills needed to create rich, interactive Java applications. This program is ideal for both beginners and experienced programmers looking to enhance their expertise in building dynamic user interfaces using JavaFX. Under the guidance of industry-experienced instructors, participants will learn to leverage JavaFX's powerful features, including scene graph-based UI components, animations, and advanced media integration. Trinity Technologies ensures that learners gain hands-on experience, making them proficient in crafting modern, responsive desktop applications, and fully prepared for the JavaFX certification exam.
Trinity Technologies, centrally located in Trivandrum, Kerala, is a leading training institute renowned for its cutting-edge IT courses. The JavaFX program at Trinity is meticulously crafted to align with industry standards, providing participants with the knowledge and skills necessary to excel in the field of Java development. With a focus on practical learning, Trinity ensures that students are well-versed in both the theoretical aspects and real-world applications of JavaFX.
Trinity Technologies takes pride in its team of expert instructors who bring years of industry experience to the classroom. These professionals are adept at simplifying complex concepts and providing students with the tools they need to succeed. The curriculum is designed to cover all essential aspects of JavaFX, from basic concepts to advanced features, ensuring that participants are equipped to handle a wide range of development challenges.
Trinity Technologies offers state-of-the-art facilities, including fully equipped labs that provide the perfect environment for learning and experimentation. The hands-on training approach adopted by Trinity ensures that students gain practical experience in developing JavaFX applications, including creating complex UI elements, implementing animations, and managing multimedia content. This practical exposure is vital for building confidence and proficiency in JavaFX development.
The JavaFX training program at Trinity Technologies covers a wide range of topics essential for mastering this versatile framework. Key areas of focus include:
JavaFX Fundamentals: Introduction to JavaFX, understanding the architecture, and setting up the development environment.
UI Components and Layouts: Working with various UI controls, layout managers, and creating responsive designs.
Graphics and Media: Integrating graphics, images, audio, and video into JavaFX applications.
Event Handling and Animations: Implementing event-driven programming and creating engaging animations.
Advanced Features: Exploring advanced concepts such as FXML, custom components, and integrating JavaFX with databases.
Deployment: Best practices for packaging and deploying JavaFX applications across different platforms.
To ensure that students are thoroughly prepared for the JavaFX certification exam, Trinity Technologies conducts regular assessments and mock tests. These evaluations help students gauge their understanding and readiness, while also familiarizing them with the exam format. The detailed feedback provided after each assessment helps identify areas for improvement, ensuring that students are fully prepared for certification.
Trinity Technologies goes beyond training by offering robust career support services. Students receive guidance on resume building, interview preparation, and job search strategies tailored to the software development industry. Trinity's strong ties with leading tech companies provide students with excellent placement opportunities, helping them secure rewarding roles as JavaFX developers, software engineers, or UI/UX designers.
Obtaining a JavaFX certification is a valuable asset for developers, signifying their expertise in creating sophisticated, high-performance desktop applications. Here's why a JavaFX certification can be a game- changer for your career:
Industry Recognition: JavaFX is widely recognized in the tech industry, and certification proves your proficiency in using this powerful framework.
Career Advancement: Certified JavaFX developers are in demand, and this certification can help you qualify for advanced roles in software development.
Skill Validation: Certification provides official recognition of your skills, making you a more attractive candidate to potential employers.
Competitive Edge: In a competitive job market, a JavaFX certification can set you apart from other candidates, enhancing your job prospects.
Trinity Technologies in Trivandrum offers a well-rounded, practical, and industry-aligned JavaFX training program that is designed to meet the needs of aspiring developers. Whether you're just starting out or looking to enhance your skills, Trinity provides the ideal platform to achieve your goals and advance your career in Java development.
What is JavaFX?
JavaFX is a software platform for creating and delivering rich Internet applications (RIAs) that can run across a wide variety of devices. It is a part of the Java platform and allows developers to create modern, visually rich user interfaces for desktop, mobile, and embedded systems using Java.
How do I get started with JavaFX development?
We will provide you with step-by-step instructions for setting up your JavaFX development environment. Additionally, we'll cover basic concepts and provide examples to help you get started.
How will JavaFX skills benefit me in the job market?
JavaFX skills are valuable for positions involving desktop application development. They also demonstrate proficiency in modern Java technologies, which can enhance your overall programming expertise and marketability.
Can I use JavaFX for mobile or web applications?
JavaFX is primarily designed for desktop applications. For mobile or web applications, different technologies such as JavaFXPorts or alternative frameworks may be considered.
Will the course include hands-on projects with JavaFX?
Yes, the course will include practical projects where you will use JavaFX to build desktop applications. These projects will help reinforce your learning and provide real-world experience.
What are the prerequisites for learning JavaFX?
Basic knowledge of Java programming is required. Understanding of object-oriented principles and previous experience with Swing or other GUI frameworks can be helpful but is not necessary.
How does JavaFX differ from Swing?
While both JavaFX and Swing are used for building graphical user interfaces in Java, JavaFX offers a more modern, flexible approach with advanced graphics capabilities, CSS styling, and better support for animations and multimedia.